Project: Health Hub
Hello there! Welcome to my project portfolio! This portfolio contains a list of contributions towards my CS2103T - Software Engineering module project, which requires me to work in a team to morph an existing functional product to something else that solves a real-world problem.
Health Hub is a desktop application that aims to get rid of the messy system of palliative care requests for health administrative staff. Admin staff who are in-charge of assigning requests from patients to qualified health staff are often overwhelmed by the enormous filings and binders that take up so much of their time.
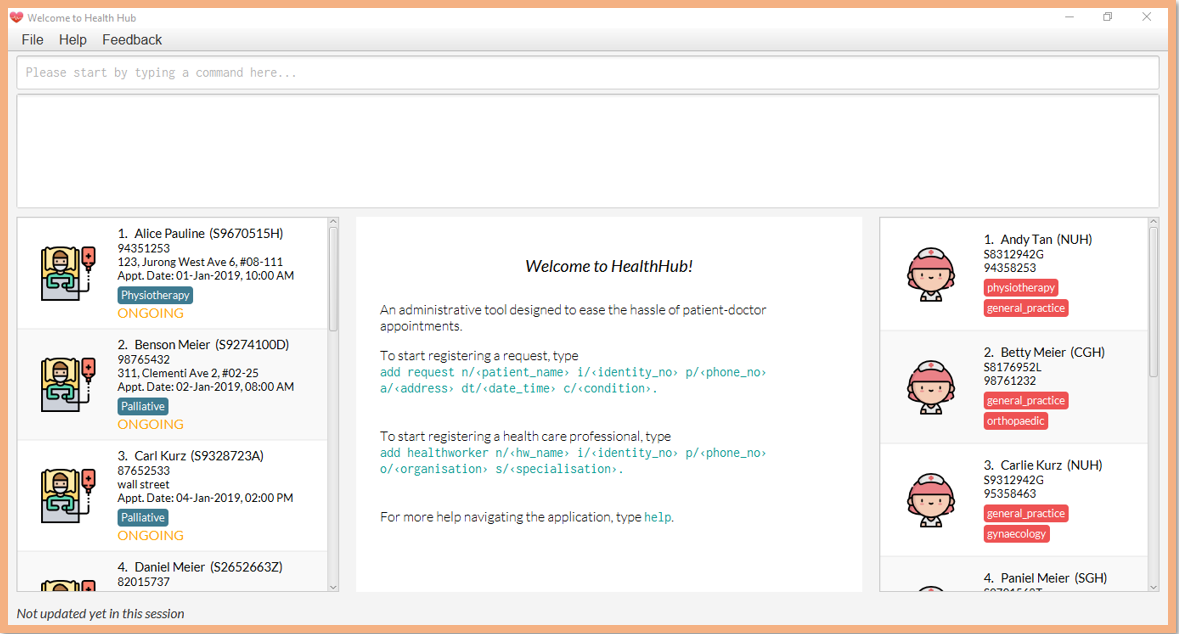
My role in the team is to handle all the User Interface (UI) coding to render model data to display it to our users, who are hospital administrative staff. Thinking that this is not enough to ensure a pleasant experience for our users, I extended some of the original functionalities from AddressBook4 and added a new command. Tests in the form of component, integration and system tests are also added to ensure quality control.
Overview
-
Restructured most of the
.fxml
files associated with the UI components -
Wrote code to render the model data into the different UI components
-
Wrote my own version of stylesheet to use for the application
-
Handle logic for displaying a request’s details in the
InfoPanel
, which is the center panel of the app. -
Extended the normal textfield to an additional autocomplete functionality.
-
Added a
setreminder
command
Summary of Contributions
-
Major Enhancement 1: restructured the original AB4 UI code into different code that will suit the context of our application.
-
What it does: Shows the lists of requests and health workers in two different panels. The UI has a center panel that displays a request’s additional details that are not visible within the list, such as the health staff assigned, and a static map overview of the patient’s address.
-
Justification: This is important as it is the interface that the user will be interacting with. Important details such as a patient’s name and NRIC are displayed differently compared to the other details such as phone or address. Special noteworthy details such as the conditions of a patient or the skills of a health worker are also displayed differently.
-
Highlights: Coding the UI requires a deep understanding of how different UI components interact with each other and how the model data is rendered to the UI layer (via
ObservableValue<Object>
). -
Credits: Myself, but integrated a UI library called
font-awesome
in the application itself to render the display of icons related to request details. The static map is provided bygothere.sg
.
-
-
Major Enhancement 2: added the ability for the user to select from a list of suggested commands, which is also known as an autocomplete feature.
-
What it does: Shows a list of suggested commands based on the character that the user has typed in the text field.
-
Justification: This is important as new users might be lost as to what kind of commands they can use. By having an autocomplete feature, they spend shorter time rewriting the same commands (e.g. adding 10 requests).
-
Highlights: Since the
CommandBox
which contains theAutoCompleteTextField
, is always used in the test cases, this new implementation affects the behaviour of existing test cases, especially the UI component and systemtests and requires a thorough rework to resolve them. -
Credits: Myself, but had read some tutorials online on how to do it.
-
-
Minor Enhancement: added a
setreminder
command to further enhance the app’s capability, by reminding the user of a task he or she needs to do within the day. -
Code Contributed: [Test code]
-
Other Contributions:
-
Project Management:
-
Enhancements to existing features:
-
Community:
-
Contributions to the User Guide
The sections below demonstrate how I used an emphathetic approach to educate the users of Health Hub on its features and future releases. |
v2.0 Release Notes
After the release of v1.4, the team have planned for future enhancements to Health Hub to improve its existing functionalities, by also considering different aspects such as the ethical part of handling sensitive patient information within and outside of the app.
For data management, it will be upgraded to an even higher level of encryption by professional standards to ensure that sensitive information is handled cautiously to prevent data leaks.
For account management, hospital administrative staff will have to start logging in to their accounts (accounts which are given to them when they first joined the department) in order to use the app. These accounts are created and managed by a higher authority personnel, to add a second layer of security.
So far, with the releases of v1.2 to v1.4, the focus is on registering the requests into the system and assigning them. In v2.0, there will be additional details added to health workers such as their availability to visit the home care patients, that will add value to when the user is assigning an open request.
Last but not least, it is important that we gather our users' feedback from time to time to understand their frustrations and needs with the app. As such, there will be a "Feedback" option in v2.0 for users to submit their feedback to the developers of Health Hub.
Setting Reminder Alerts: setreminder
Sometimes when you have too many tasks at hand, it can be quite cumbersome to remember what tasks you need to complete for the day. You might want to phone up a patient to remind him of an appointment before the health worker visits his home, because you recall that this patient was not at home the last time the health worker visited. The phone call from you can prepare him to be at his home when the health worker arrives, so he can provide the necessary health care services. It can also remind you when it is lunch time, so you can avoid the peak period!
Format: setreminder t/<hh:mm:ss> m/<customised_message>
Using the setreminder
command, you can set a reminder alert to notify you at a certain time of the day, with your
own message that you can customise to remind yourself.
The alert only shows for when Health Hub is running in the background process. |
In the example below, a reminder has been set to notify the user at 6.30PM with the customised message to "to call patient Tan Ah Hock for his appointment later at night".
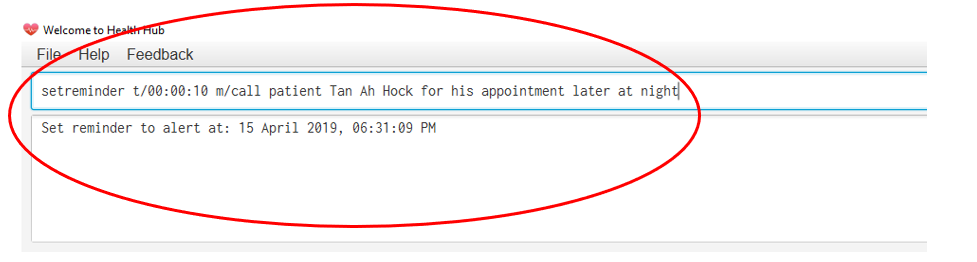
Figure 5.14.1 shows the format of the setreminder
command in the text field.
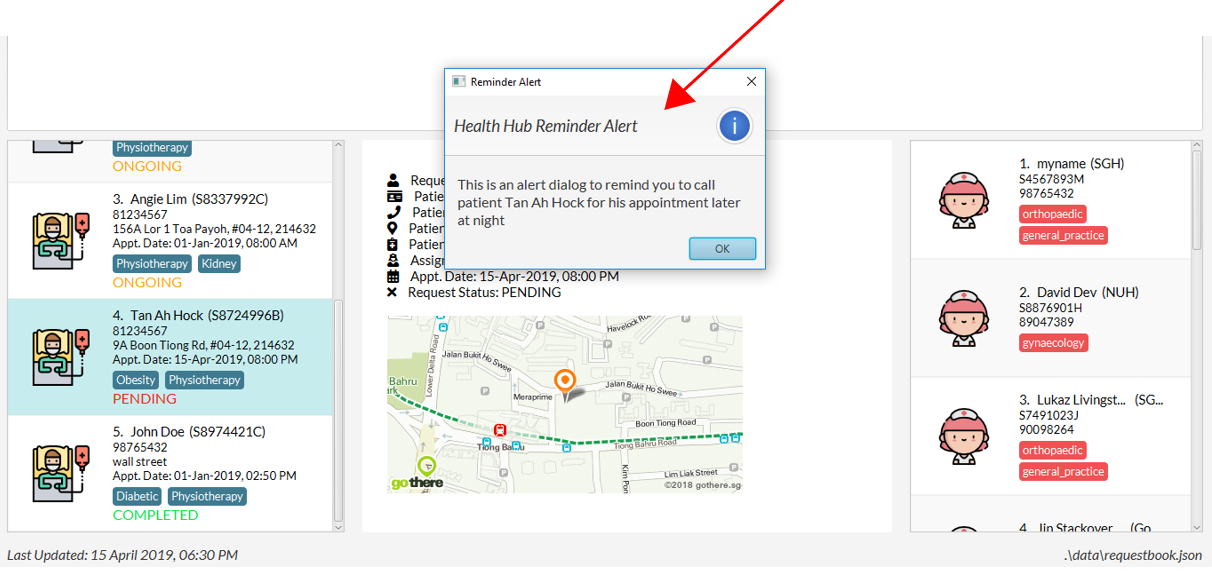
Figure 5.14.2 shows the result of the reminder alert in the GUI after it is time for the app to notify the user.
In addition to the sections above, I also formatted the Command Summary table in the user guide in a neat manner so users are able to look for the key information for the different commands. |
Contributions to the Developer Guide
The sections below showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
UI component
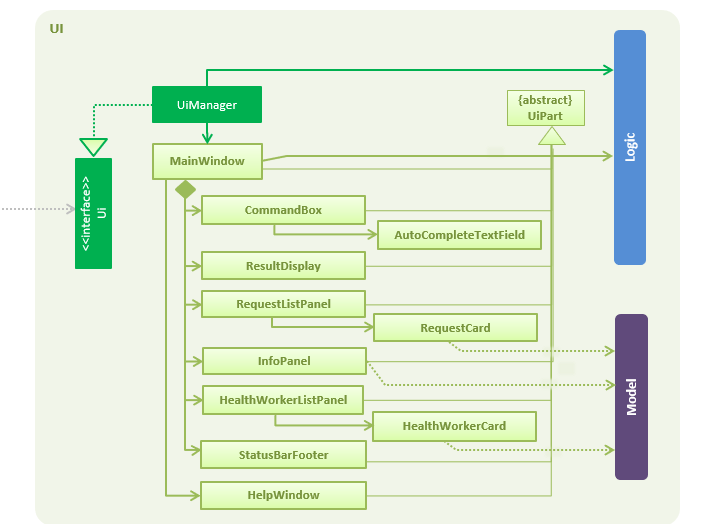
API : UI Package
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, RequestListPanel
, InfoPanel
, HealthWorkerListPanel
, StatusBarFooter
, etc.
All of these, including the MainWindow
, inherit from the UiPart
class, which is an abstract class containing methods for handling the loading of FXML
files.
The UI
component uses the JavaFX UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Listens for changes to the
Model
data so that the UI can be updated with the modified data dynamically.
Set Reminder Feature
The setreminder
command gives the user an option to set a reminder with a customised message.
The reminder is in the form of an AlertDialog
and is scheduled to display on the UI by a TimerTask
at time
t + current time
, where t
is time in the format hh:mm:ss
.
Current Implementation
The following sequence diagram shows the sequence flow from when the SetReminderCommandParser
receives the command and
parses it into a SetReminderCommand
object.
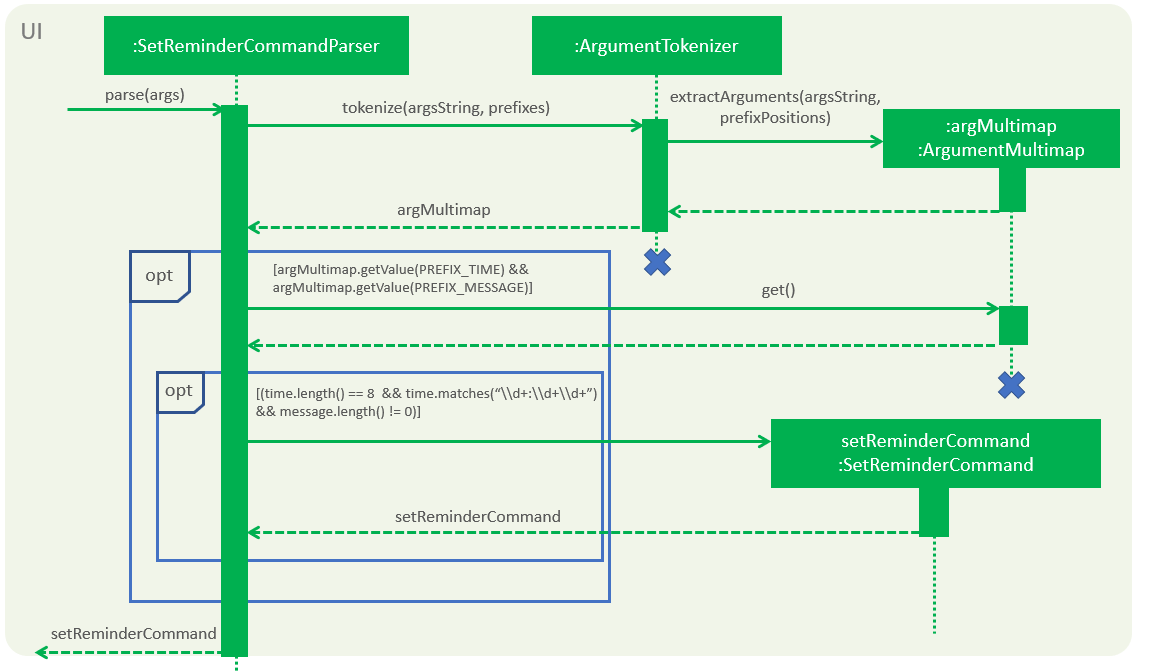
Figure 3.9.1 shows the sequence diagram for the different method invocation calls between the classes
-
When
SetReminderCommandParser
receives theparse
command, it calls the static methodArgumentTokenizer.tokenize
to extract the arguments from the user input string. -
The
extractArguments
constructs and returns anArgumentMultimap
based on the arguments passed in. -
SetReminderCommandParser
checks if there are arguments specified under prefixesPREFIX_TIME
andPREFIX_MESSAGE
. -
If it cannot find those arguments, it throws a
ParseException
to the calling program. -
If arguments are available, it calls the
getValue
method ofArgumentMultimap
to return the values -
After getting the values, the
SetReminderCommandParser
checks for the validity of the values and if true, calls the constructor ofSetReminderCommand
Autocomplete Feature for CommandBox
The UI component, CommandBox
, comes with a TextField
component in AB4. For Health Hub, the TextField
is replaced
with an AutoCompleteTextField
class that extends from the parent class, TextField
.
In the AutoCompleteTextField
class, it adds an additional listener to the textProperty()
of TextField
class, to register for when
a text input is being sensed and generates the suggestion box in the form of a ContextMenu
to the user.
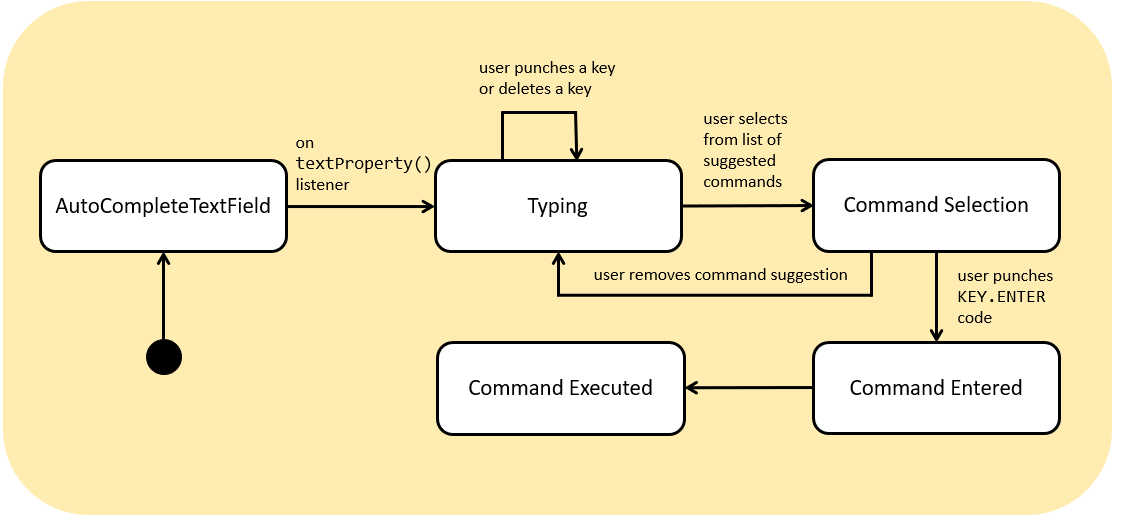
Figure 3.11.1 shows the state machine diagram for the AutoCompleteTextField
behaviour when it listens for a user input.
The searching of which commands to display to the user as suggestions is done by iterating the suggestions
data structure, which is a TreeSet
, then checking
against the user’s input if any of the suggestions starts with the user’s text.
Aspect: Data structure to store the list of commands
Implementation | Use a TreeSet data structure |
Use a SuffixTree data structure |
---|---|---|
Pros |
Implements the |
Made for features like autocompletion, allows particularly fast implementation of |
Cons |
Elements contained within the |
Suffix Trees are difficult to comprehend → difficult to implement. |